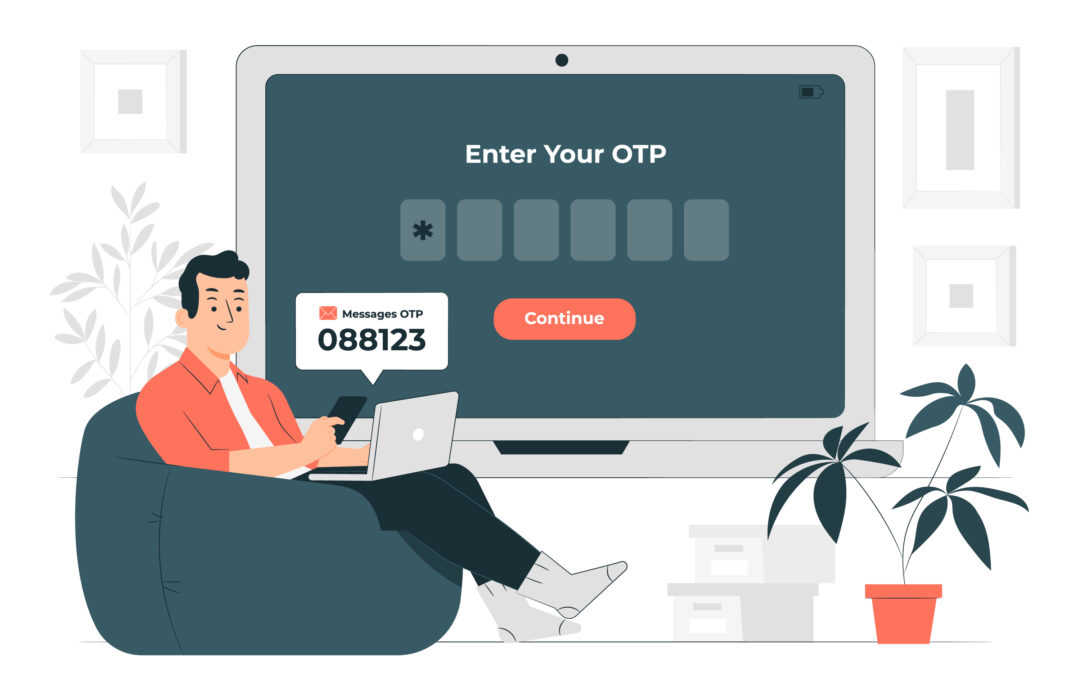
Creating an OTP Generator Program: A Step-by-Step Guide
When creating an OTP generator program, it is essential to understand the underlying principles and algorithms behind it. The first step is to decide on the type of OTP generator to implement. There are several options available, including time-based OTPs (TOTPs) and event-based OTPs (HOTPs).
If you choose to implement a TOTP, you will need to use a cryptographic hash function, such as SHA-1 or SHA-256, to generate the OTP. TOTPs are time-based, meaning that the generated OTP changes periodically, typically every 30 seconds. To achieve this, you will need to incorporate the current time into the OTP generation process. This can be done by converting the current time into a timestamp and dividing it by a predefined time step, such as 30 seconds.
On the other hand, if you decide to implement an HOTP, you will need to use a counter-based approach. HOTPs generate a unique OTP for each login attempt, incrementing a counter value each time. The OTP is derived from the counter value using a cryptographic hash function. This ensures that each OTP is unique and cannot be predicted without knowledge of the counter value.
Regardless of the type of OTP generator you choose to implement, it is crucial to ensure the security of the generated OTPs. This can be achieved by using a strong cryptographic hash function and storing the secret key securely. It is also recommended to enforce additional security measures, such as rate limiting and account lockouts, to protect against brute-force attacks.
Once you have decided on the type of OTP generator and implemented the necessary algorithms, you can integrate it into your application. This typically involves adding a step in the login process where the user is prompted to enter the OTP generated by the program. The entered OTP is then compared to the expected OTP generated by the program. If they match, the user is granted access; otherwise, access is denied.
In conclusion, creating an OTP generator program requires a thorough understanding of the underlying principles and algorithms. By implementing a secure and reliable OTP generator, you can enhance the security of your application and protect user accounts from unauthorized access.
Understanding OTP Generation
Before diving into the implementation details, let’s understand the basic concept behind OTP generation. OTPs are time-based or event-based passwords that are valid for a short period of time or a single use. They are typically generated using a combination of a secret key and a counter or timestamp.
The OTP generation algorithm involves several steps:
- Generate a secret key: A secret key is a random string of characters that is known only to the user and the server. This key is used to generate the OTP.
- Generate a counter or timestamp: The counter or timestamp is used to ensure that each OTP is unique. It can be based on the current time or incremented with each new OTP generation.
- Combine the secret key and counter/timestamp: The secret key and counter/timestamp are combined using a cryptographic function, such as HMAC-SHA1, to generate a unique OTP.
- Truncate the OTP: The generated OTP is usually a long string of characters. To make it more user-friendly, it is truncated to a fixed length (e.g., 6 digits).
Once the OTP is generated, it can be sent to the user via a secure channel, such as SMS or email. The user can then enter the OTP into the authentication system to verify their identity. Since OTPs are time-based or event-based, they provide an additional layer of security compared to traditional static passwords.
It’s important to note that the secret key used for OTP generation should be kept secure and not shared with anyone. If an attacker gains access to the secret key, they can generate valid OTPs and potentially bypass the authentication system.
In addition to the basic OTP generation algorithm described above, there are also variations that can be used to enhance security. For example, some systems use a rolling window of OTPs, where multiple OTPs are generated in advance and stored on both the server and the user’s device. This allows for offline OTP verification and reduces the reliance on a real-time clock.
Overall, OTP generation is a crucial component of modern authentication systems, providing an extra layer of security to protect against unauthorized access. By understanding the underlying concepts and implementing best practices, organizations can ensure the integrity and confidentiality of their systems and data.
Algorithm Structure
Now that we have a basic understanding of OTP generation, let’s outline the algorithm structure for creating an OTP generator program:
- Define the secret key: Start by defining a secret key that will be used to generate the OTP. This key should be kept secure and not shared with anyone. The secret key can be a randomly generated string of characters, such as a combination of letters, numbers, and special symbols. It is important to choose a strong secret key to ensure the security of the OTP.
- Generate a counter or timestamp: Depending on the type of OTP generation (time-based or event-based), generate a counter or timestamp value. For time-based OTPs, you can use the current time in seconds since the epoch. This can be obtained using a programming language’s built-in functions or libraries. For event-based OTPs, you can use a counter that increments with each OTP generation. This counter can be stored in a database or memory for future use.
- Combine the secret key and counter/timestamp: Use a cryptographic function, such as HMAC-SHA1, to combine the secret key and the counter/timestamp value. This will generate a unique OTP. The cryptographic function takes the secret key and the counter/timestamp as input and produces a hash value. The hash value is a fixed-length string that is unique for each combination of secret key and counter/timestamp.
- Truncate the OTP: To make the OTP more user-friendly, truncate it to a fixed length (e.g., 6 digits) by taking a substring of the generated OTP. This can be done by selecting the first 6 characters of the OTP or by using a specific truncation algorithm. Truncating the OTP reduces its length and makes it easier for users to enter or remember.
- Display or use the OTP: Finally, display the generated OTP to the user or use it for further authentication or verification purposes. The OTP can be displayed on a web page, mobile app, or any other user interface. It can also be used for two-factor authentication, where the user is required to enter both their password and the OTP to access a secure system or perform a sensitive operation.
By following this algorithm structure, you can create a robust and secure OTP generator program that can be used in various applications, such as online banking, email verification, or secure login systems. The secret key and the cryptographic functions ensure the uniqueness and randomness of the OTP, while the truncation and display steps make it user-friendly and easy to use. Overall, OTP generation is a crucial aspect of modern security systems, providing an additional layer of protection against unauthorized access and identity theft.
To further enhance the security of the OTP generator program, we can implement additional features such as user input validation and error handling. This will ensure that the program can handle various scenarios and provide a smooth user experience.
One way to implement user input validation is by checking the length and format of the secret key. The secret key should be a string of alphanumeric characters and have a minimum length of, let’s say, 8 characters. We can use regular expressions to validate the secret key before generating the OTP.
Additionally, we can implement error handling to handle any unexpected errors that may occur during the execution of the program. For example, if there is an issue with the HMAC-SHA1 hash generation or if the OTP cannot be generated, we can display an error message to the user and prompt them to try again.
Furthermore, we can add a feature to allow the user to specify the length of the OTP they want to generate. By default, we are currently generating a 6-digit OTP, but some applications may require longer or shorter OTPs. We can modify the `generate_otp` function to accept an optional parameter for the OTP length and update the truncation step accordingly.
Another improvement we can make is to implement a mechanism to store and manage the counter value. Currently, we are using the current timestamp as the counter, but in a real-world scenario, we would need a more robust and secure way to manage the counter value. We can consider using a database or a file system to store and retrieve the counter value, ensuring that it is incremented correctly for each OTP generation.
In conclusion, by implementing user input validation, error handling, customizable OTP length, and a counter management mechanism, we can enhance the security and functionality of the OTP generator program. These additional features will provide a more reliable and user-friendly experience for generating OTPs.