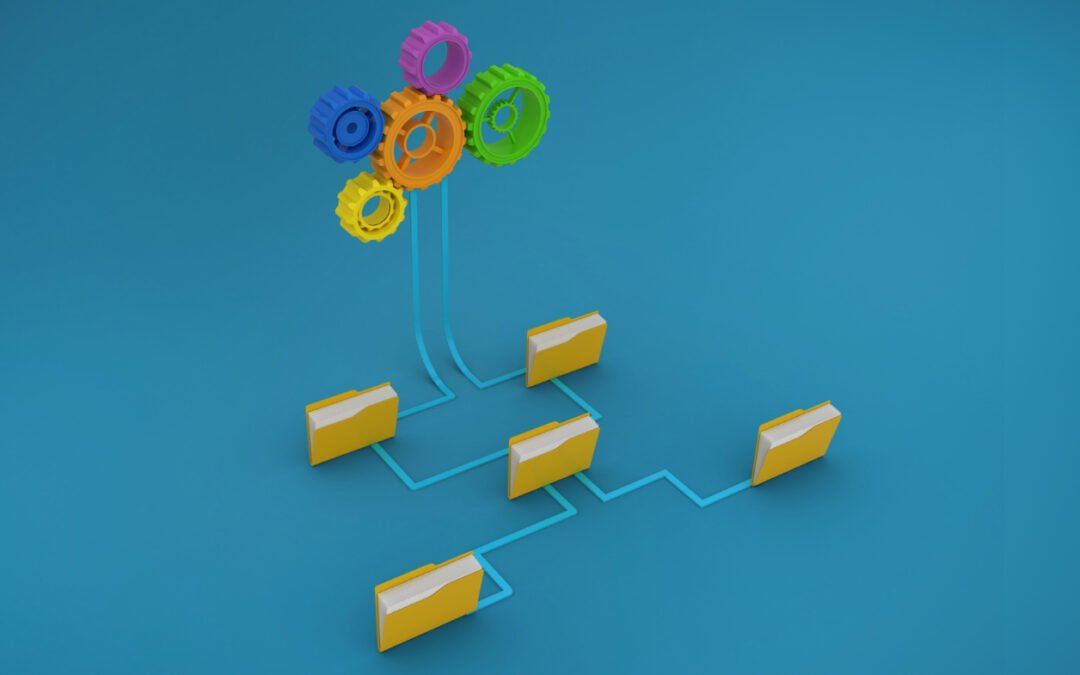
Unraveling the Mysteries of Sorting Algorithms
Introduction:
Sorting algorithms are the bread and butter of computer science and play a crucial role in various applications across industries. These algorithms arrange a list or array of elements in a specific order—ascending or descending. From the everyday task of alphabetizing a list of names to more complex applications like database query optimizations, sorting is omnipresent.
Importance of Sorting:
- Efficient Data Retrieval: Efficiently sorted data can speed up search operations like binary search, where the data being searched for is compared against the middle element.
- Data Visualization: Sorted data can lead to better insights when visualized, making patterns and anomalies easily identifiable.
- Optimization Algorithms: Many algorithms, such as those used in computer graphics, rely on sorted data for optimal performance.
Classification of Sorting Algorithms:
Sorting algorithms can be broadly classified based on the following:
- Time Complexity: Some algorithms work well for small datasets (e.g., Bubble Sort), while others are better for large datasets (e.g., Merge Sort).
- Space Complexity: Algorithms might use extra space (like Merge Sort) or sort in place (like QuickSort).
- Stability: A stable sorting algorithm will maintain relative order if two elements have equal values.
- Internal vs. External Sorting: Internal sorting happens entirely in the main memory. In contrast, external sorting uses external storage, suitable for sorting massive data volumes that don’t fit in memory.
Common Sorting Algorithms:
- Bubble Sort:
A simple comparison-based algorithm where the list is iterated multiple times, swapping adjacent elements if they are in the wrong order. Its average and worst-case time complexity is .
- Insertion Sort:
It builds the final sorted list one item at a time. It is much less efficient on larger lists than more advanced algorithms like quicksort, heapsort, or merge sort, with an average time complexity of (O(n^2)).
- Selection Sort:
The main idea behind the algorithm is to find the smallest (or largest) element from the unsorted sublist and swap it with the leftmost unsorted element, moving the sublist boundaries one element to the right. It has a time complexity of (O(n^2)).
- Merge Sort:
It’s a ‘divide and conquer’ algorithm that splits an array in half, recursively sorts the halves, and then merges them. While it has a time complexity of (O(n log n)) in all cases, it requires (O(n)) extra space.
- QuickSort:
Another ‘divide and conquer’ method selects a ‘pivot’ element and partitions the array, putting all more minor elements before and larger ones after the pivot. Then, it recursively sorts the sub-arrays. While its average case time complexity is (O(n log n)), it can degrade to (O(n^2)) in the worst case.
- HeapSort:
This algorithm leverages a binary heap data structure. It works by visualizing the data as a nearly complete binary tree, then repeatedly extracts the maximum element from the heap and reconstructs the heap. It has a time complexity of \(O(n \log n)\).
Modern Sorting Algorithms:
- Timsort: Derived from merge sort and insertion sort, it’s designed to perform well on real-world data and is the default sorting algorithm in Java’s `Arrays. sort()` and Python’s `sorted().`
- Introsort: A hybrid sorting algorithm that provides fast average and optimal worst-case performance. It begins with quicksort, switches to heapsort when the recursion depth exceeds a certain level, and switches to insertion sort for small-sized arrays.
Conclusion:
Sorting algorithms, with their wide range of applications, are an integral part of algorithmic studies. The choice of a sorting algorithm often depends on a task’s specific requirements, including the dataset’s size, available memory, and desired stability. Understanding these algorithms’ underlying principles and characteristics aids in making informed decisions in software development and computational tasks.